YourDyno Plugin system
YourDyno’s functionality can be extended by plugins. Plugins are pieces of code written in a .Net language like C# or Visual Basic.
You find YourDyno’s plugin library here.
Anyone with programming skills can write their own plugins and we encourage everyone who has made a good working plugin to upload it to our library for everyone to use. Power to the People, right ;-)!
Installing plugins
New versions of YourDyno include all available plugins, and you can choose during installation which ones to install. You can run the installer again later to add or remove plugins from this predefined list by choosing Modify when the installer prompts.
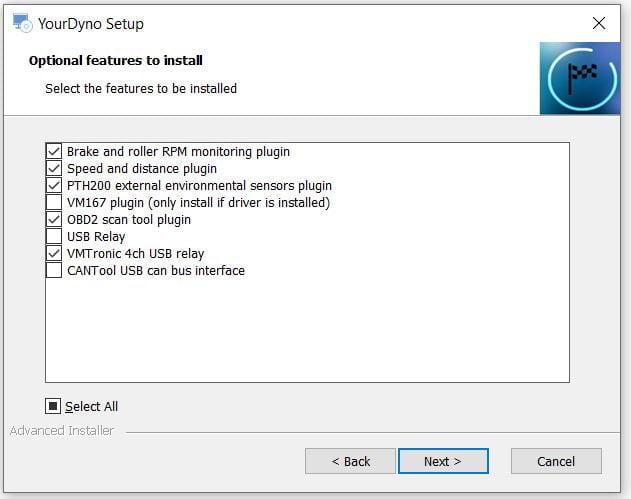
Description of the plugins are in the library. It is recommended to always install the standard plugins using the installer. The library also includes the source code for some plugins.
To manually install your own or a 3rd party plugin, open “Manage Plugins” in YourDyno software, press “Install plugin…” and select the .dll file you downloaded. The plugin in installed. Verify that the plugin appears in the “Installed plugins” list.
You can also copy the .dll file directly to the plugin directory, which is located at %ProgramData%\YourDynoPlugins (this is normally C:\ProgramData\YourDynoPlugins).
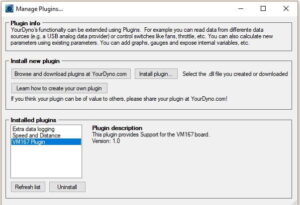
Removing or upgrading plugins
Use the installer, click Modify.
Or manually like this: You need to delete the .dll file from your
%ProgramData%\YourDynoPlugins directory to uninstall a plugin. To upgrade you can just copy the new .dll over the old .dll, or delete the file, then open YourDyno and do a new install using the new .dll
Debugging plugin installation issues
If you have issues installing plugins or your get errors when starting YourDyno, you can remove all plugins (move the .dlls to another directory) and then add them back one by one to see which one is acting up.
Making your own plugin
Plugins are made using the Managed Extensibility Framework (MEF) system in Visual Studio. Here is a description of it, but it is not necessary to understand it all to write a plugin.
The plugins are stored in a the directory %ProgramData%\YourDynoPlugins (typically C:\ProgramData\YourDynoPlugins). The plugin is just a .dll file that YourDyno parses and instantiates.
Each plugin implements an interface defined by YourDyno’s PluginContracts library (.dll). Here is the interface:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using DataConnection; using System.Windows; using System.Windows.Forms; namespace PluginContracts { public enum UnitType // used when switching units { Temperature = 1, Power = 2, Torque = 3, Other = 4, None = 0 } public class OnePlugInDataConnection { public string pluginName; // name of the plugin (same as 'name' in IModule and IDataIOProvider) public float y; // polled data public int graphPane; // 1 = 1st graph pane, 2 = 2nd graph pane, 3 = 3rd graph pane (0 = off is depreciated) public bool isY2Axis; // whether to use the left or right (Y2) axis in the graphs public bool showGaugeInRunWindow; public string name; // Name is used to identify gauges and graphs public string unit; // the unit that the data is measured in (e.g. N, kW, C, K, etc). Can be empty ("") public bool applyNoiseFiltering; // if true, noise filtering will be applied public UnitType unitType; } // The main plugin interface. Can implement a data I/O provider, for example a USB analog input/output device or a USB relay controller // Can also provide logging of internal parameters // Data inputs will be polled at the same data rate as other data // Plugin can implement logic to turn on/off its outputs based on data from dynoDataConnection and user settings public interface IDataIOProvider { string name { get; } string pluginDescription { get; } string version { get; } List<System.Windows.Forms.Keys> hotkeys { get; } // list your hotkeys here void hotkeyPressed(Keys hotkey); // YourDyno will call this function when a hotkey is pressed void initDynoDataConnection(DynoDataConnection dynoDataConnection, List<OnePlugInDataConnection> allPlugins); // access to data from dyno box and other plugins List<OnePlugInDataConnection> pluginDataConnections { get; } // the data that the plugin provides to YourDyno System.Windows.Forms.ToolStripMenuItem pluginMenuEntry { get; } event ConfigChangeEventHandler OnConfigurationChange; // trigger this event to update configuration (i.e. signal names, etc) } public delegate void ConfigChangeEventHandler(object source, ConnectionChangeEventArgs e); public class ConnectionChangeEventArgs : EventArgs { public List<OnePlugInDataConnection> pluginDataConnections; } }
In your Visual Studio project you need to add the .dll’s from the YourDyno install directory as needed. Take a look at the example plugins to get going.